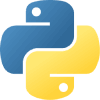
Automate tasks with Python
Execute Python code within your automated task.
https://www.python.org
Actions
The action is what happens automatically, without you having to do anything else.
-
Execute a Python script
Run a Python script as part of your task.
Frequently Asked Questions
How do I provide my Python script code to Botize?
To make Botize execute your Python script as part of an automated task, you must:
1. Create your Python code.
2. Upload it to a server to make it accessible via a public URL.
3. Provide that URL to Botize in the corresponding step of your task.
Botize will execute your script and automatically pass the values generated in previous steps to your code.
What Python libraries can I use in Botize?
Botize includes several standard Python libraries you can use in your scripts, such as:
- json: for handling JSON data.
- requests: for making HTTP requests.
- datetime: for working with dates and times.
You cannot install or use external libraries. You only have access to the ones provided by default.
What is the most basic code to get started?
Here's a simple example of a script that generates a new tag:output_data = {"message": "Hello from Python"}
This code creates a tag named message with the value "Hello from Python", which will be available for the following steps of your task.
How can I access task tags from my Python code?
All the tags generated in previous steps of your task are available in the input_data variable. It’s a key-value array, where the key is the tag name and the value is its content.
Example of access:my_tag = input_data["tag_name"]
How do I modify or create new tags in my Python code?
To create new tags or modify existing ones, use the output_data variable. Any data you store there will be available as tags in the following steps of the task.
Example: Modifying an existing tag and creating a new one:lowercase_text = input_data["text"].lower()
output_data = {"lowercase_text": lowercase_text, "message": "Tag generated successfully"}
How can I test my Python script before using it in Botize?
You can test your script locally by simulating the content of input_data as a Python dictionary:input_data = {"text": "THIS IS AN EXAMPLE"}
lowercase_text = input_data["text"].lower()
output_data = {"lowercase_text": lowercase_text}
print(output_data)
Once it works, upload it to your server and provide the URL to Botize.
What happens if my script has an error?
If your script generates an error during execution, Botize will display a message indicating something went wrong. Make sure to handle potential errors in your code using try/except blocks:try:
lowercase_text = input_data["text"].lower()
output_data = {"lowercase_text": lowercase_text}
except KeyError as e:
output_data = {"error": f"Tag not found: {e}"}
In any case, if your script fails, Botize will return the tags 'errorMessage', 'errorType', and 'stackTrace' with enough information to help you identify the error, its type, and location.
Can I make requests to external APIs from my Python script?
Yes, you can use the requests library to interact with external APIs. For example:import requests
response = requests.get("https://api.example.com/data")
if response.status_code == 200:
data = response.json()
output_data = {"api_result": data}
else:
output_data = {"error": f"API error: {response.status_code}"}
Can I use Python scripts for complex calculations or transformations?
Yes, as long as you use the included libraries. For example, you can handle dates using the datetime library:from datetime import datetime
current_date = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
output_data = {"current_date": current_date}
What limitations should I be aware of?
- You cannot install additional libraries.
- The execution time of your script must be reasonable (avoid slow operations).
- Make sure your script’s URL is public and accessible from the internet.